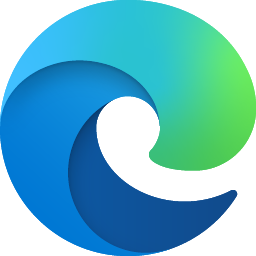
Hi @mnxl ,
The snippet you shared works well for me. For this particular error, you may need to add these command lines:
edge_options.add_argument("--disable-dev-shm-usage")
edge_options.add_argument("--no-sandbox")
You can also try other ports if the error is still thrown.
For the browser version WebView2 app is using, just use print(edge_driver.capabilities['browserVersion'])
to check the version, and then use a compatible Edge driver for it.
UPDATE
I have reproduced this issue. The cause is another WebView2 app has already been launched prior to this app that is meant to be connected to. For example, Outlook desktop app uses WebView2 component, so if I launched Outlook desktop app before my own WebView2 app, the driver will not be able to connect to my own app.
2 solutions to this problem:
- Prevent any WebView2 app being launched before your target app.
- (Recommended) Don't set the environment variable globally (in the system settings). Set the environment variable only for your target application.
To set the environment variable per-app, following example is for reference
var options = new CoreWebView2EnvironmentOptions();
options.AdditionalBrowserArguments = "--remote-debugging-port=9222";
var env = await CoreWebView2Environment.CreateAsync(null, null, options);
await webView.EnsureCoreWebView2Async(env);
If the answer is helpful, please click "Accept Answer" and kindly upvote it. If you have extra questions about this answer, please click "Comment".
Note: Please follow the steps in our documentation to enable e-mail notifications if you want to receive the related email notification for this thread.
Best Regards,
Shijie Li