진행률 표시기 - MRTK2
예제 장면
진행률 표시기를 사용하는 방법의 예는 장면에서 찾을 ProgressIndicatorExamples
수 있습니다. 이 장면에서는 SDK에 포함된 각 진행률 표시기 프리팹을 보여 줍니다. 또한 장면 로드와 같은 몇 가지 일반적인 비동기 작업과 함께 진행률 표시기를 사용하는 방법을 보여 줍니다.
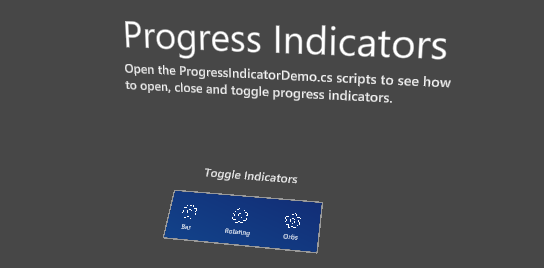
예: 진행률 표시기 열기, 업데이트 & 닫기
진행률 표시기가 인터페이스를 구현합니다 IProgressIndicator
. 이 인터페이스는 를 사용하여 GetComponent
GameObject에서 검색할 수 있습니다.
[SerializedField]
private GameObject indicatorObject;
private IProgressIndicator indicator;
private void Start()
{
indicator = indicatorObject.GetComponent<IProgressIndicator>();
}
및 메서드는 IProgressIndicator.OpenAsync()
작업을 반환합니다.IProgressIndicator.CloseAsync()
비동기 메서드에서 이러한 작업을 기다리는 것이 좋습니다.
장면에 배치할 때 MRTK의 기본 진행률 표시기 프리팹이 비활성 상태여야 합니다. 메서드 IProgressIndicator.OpenAsync()
를 호출하면 진행률 표시기가 자동으로 게임 개체를 활성화하고 비활성화합니다. (이 패턴은 IProgressIndicator 인터페이스의 요구 사항이 아닙니다.)
표시기 Progress
속성을 0-1의 값으로 설정하여 표시된 진행률을 업데이트합니다. 표시된 메시지를 업데이트하려면 해당 Message
속성을 설정합니다. 구현이 다르면 이 콘텐츠가 다른 방식으로 표시할 수 있습니다.
private async void OpenProgressIndicator()
{
await indicator.OpenAsync();
float progress = 0;
while (progress < 1)
{
progress += Time.deltaTime;
indicator.Message = "Loading...";
indicator.Progress = progress;
await Task.Yield();
}
await indicator.CloseAsync();
}
표시기 상태
표시기 State
속성은 유효한 작업을 결정합니다. 잘못된 메서드를 호출하면 일반적으로 표시기가 오류를 보고하고 아무 작업도 수행하지 않습니다.
시스템 상태 | 유효한 작업 |
---|---|
ProgressIndicatorState.Opening |
AwaitTransitionAsync() |
ProgressIndicatorState.Open |
CloseAsync() |
ProgressIndicatorState.Closing |
AwaitTransitionAsync() |
ProgressIndicatorState.Closed |
OpenAsync() |
AwaitTransitionAsync()
은 표시기를 사용하기 전에 표시기가 완전히 열리거나 닫혀 있는지 확인하는 데 사용할 수 있습니다.
private async void ToggleIndicator(IProgressIndicator indicator)
{
await indicator.AwaitTransitionAsync();
switch (indicator.State)
{
case ProgressIndicatorState.Closed:
await indicator.OpenAsync();
break;
case ProgressIndicatorState.Open:
await indicator.CloseAsync();
break;
}
}