Use the combo control
This article shows samples about the combo control. There are TypeScript and JavaScript examples.
Tip
Check out our newest developer site, Formula Design System.
How to organize your code
In the home
folder for your project, create a main.html
file with the following contents:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
</head>
<body>
<script src="sdk/scripts/VSS.SDK.js"></script>
<script>
VSS.init({ usePlatformScripts: true });
VSS.ready(function() {
require(["scripts/main.js"], function () { });
});
</script>
<div class="sample-container"/>
</body>
</html>
Ensure that the VSS.SDK.js
file is inside the sdk/scripts
folder so that the path is home/sdk/scripts/VSS.SDK.js
.
Put the following code snippets into a main.js
file in a scripts
folder, so that the path is home/scripts/main.js
.
Combos
For more details, see the Combo Control API reference.
Plain list combo
This sample shows combo of type list
. It also shows how change
delegate is used to populate another combo.
If the second combo doesn't have any source, its mode is changed to text to hide the unnecessary drop icon.
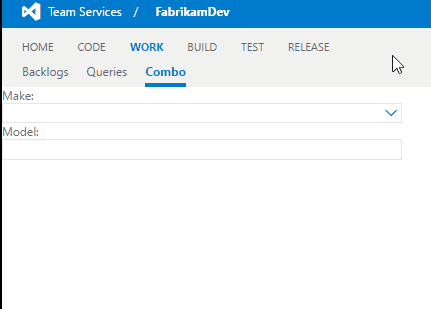
import Controls = require("VSS/Controls");
import Combos = require("VSS/Controls/Combos");
var container = $(".sample-container");
var makeOptions = <Combos.IComboOptions>{
width: "400px",
source:
["Aston Martin", "Audi (3)", "Bentley", "BMW (2)", "Bugatti",
"Ferrari", "Ford", "Honda", "Hyundai", "Kia", "Lamborghini",
"Land Rover", "Lotus", "Maserati", "Mazda", "Mercedes",
"Mitsubishi", "Nissan", "Porsche", "Toyota", "Volkswagen", "Volvo"],
change: function () {
var selected = this.getText();
if (selected.indexOf("Audi") === 0) {
modelCombo.setSource(["A3", "A4", "Q7"]);
modelCombo.setMode("drop");
}
else if (selected.indexOf("BMW") === 0) {
modelCombo.setSource(["325", "X5"]);
modelCombo.setMode("drop");
}
else {
modelCombo.setMode("text");
}
modelCombo.setText("");
}
};
var modelOptions = <Combos.IComboOptions>{
width: "400px",
mode: "text"
};
// Make combo
$("<label />").text("Make:").appendTo(container);
Controls.create(Combos.Combo, container, makeOptions);
// Model combo
$("<label />").text("Model:").appendTo(container);
var modelCombo = Controls.create(Combos.Combo, container, modelOptions);
Tree combo
This sample shows combo of type tree
which displays its source hierarchically by supporting expand/collapse. It also supports search by node.

import Controls = require("VSS/Controls");
import Combos = require("VSS/Controls/Combos");
import TreeView = require("VSS/Controls/TreeView");
var container = $(".sample-container");
var treeOptions: Combos.IComboOptions = {
type: TreeView.SearchComboTreeBehaviorName,
width: "400px",
sepChar: ">",
source: [
{
text: "wit",
children: [{ text: "platform", children: [{ text: "client" }, { text: "server" }] }, { text: "te" }]
}, {
text: "vc"
}, {
text: "webaccess", children: [{ text: "platform" }, { text: "agile" }, { text: "requirements" }]
}, {
text: "etm"
}, {
text: "build"
}
],
change: function () {
commandArea.prepend($("<div />").text(this.getText()));
}
};
$("<label />").text("Area Path:").appendTo(container);
Controls.create(Combos.Combo, container, treeOptions);
var commandArea = $("<div style='margin:10px' />").appendTo(container);
Datetime picker
This sample shows the usage of combo by type date-time
. Please note that selecting different day from the picker preserves the hour value.

import Controls = require("VSS/Controls");
import Combos = require("VSS/Controls/Combos");
var container = $(".sample-container");
var dateTimeOptions:Combos.IDateTimeComboOptions = {
value: "Tuesday, September 29, 1982 8:30:00 AM",
type: "date-time",
dateTimeFormat: "F",
width: 300,
change: function () {
commandArea.prepend($("<div />").text(dateTimeCombo.getValue<Date>().toString()));
}
};
$("<label />").text("Select your birthday:").appendTo(container);
var dateTimeCombo = Controls.create(Combos.Combo, container, dateTimeOptions);
var commandArea = $("<div style='margin:10px' />").appendTo(container);
Multivalue combo
This sample shows the usage of combo by type multi-value
.
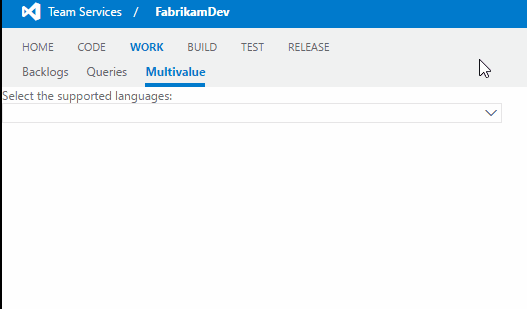
<div class="tab-content">
<div id="typescript_multivalue" class="tab-pane fade in active">
<pre><code class="lang-javascript">
import Controls = require("VSS/Controls");
import Combos = require("VSS/Controls/Combos");
var container = $(".sample-container");
var multiValueOptions: Combos.IComboOptions = {
type: "multi-value",
width: 500,
source: [
"English", "Chinese", "German", "Turkish", "Spanish",
"Japanese", "Korean", "Russian", "Portuguese", "French",
"Italian", "Arabic"],
change: function () {
// Displaying the selected value
commandArea.prepend($("<div />").text(this.getText()));
}
};
$("<label />").text("Select the supported languages:").appendTo(container);
var multiValueCombo = Controls.create(Combos.Combo, container, multiValueOptions);
var commandArea = $("<div style='margin:10px' />").appendTo(container);