ImageAttributes::SetRemapTable method (gdiplusimageattributes.h)
The ImageAttributes::SetRemapTable method sets the color-remap table for a specified category.
Syntax
Status SetRemapTable(
[in] UINT mapSize,
[in] const ColorMap *map,
[in, optional] ColorAdjustType type
);
Parameters
[in] mapSize
Type: UINT
INT that specifies the number of elements in the map array.
[in] map
Type: const ColorMap*
Pointer to an array of ColorMap structures that defines the color map.
[in, optional] type
Type: ColorAdjustType
Element of the ColorAdjustType enumeration that specifies the category for which the color-remap table is set. The default value is ColorAdjustTypeDefault.
Return value
Type: Status
If the method succeeds, it returns Ok, which is an element of the Status enumeration.
If the method fails, it returns one of the other elements of the Status enumeration.
Remarks
A color-remap table is an array of ColorMap structures. Each ColorMap structure has two Color objects: one that specifies an old color and one that specifies a corresponding new color. During rendering, any color that matches one of the old colors in the remap table is changed to the corresponding new color.
An ImageAttributes object maintains color and grayscale settings for five adjustment categories: default, bitmap, brush, pen, and text. For example, you can specify a color remap for the default category, a color-remap table for the bitmap category, and still a different color-remap table for the pen category.
The default color- and grayscale-adjustment settings apply to all categories that don't have adjustment settings of their own. For example, if you never specify any adjustment settings for the pen category, then the default settings apply to the pen category.
As soon as you specify a color- or grayscale-adjustment setting for a certain category, the default adjustment settings no longer apply to that category. For example, suppose you specify a collection of adjustment settings for the default category. If you set the color-remap table for the pen category by passing ColorAdjustTypePen to the ImageAttributes::SetRemapTable method, then none of the default adjustment settings will apply to pens.
Examples
The following example creates an Image object based on a .bmp file and then draws the image. The code creates an ImageAttributes object and sets its default remap table so that red is converted to blue. Then the code draws the image again using the color adjustment specified by the remap table.
VOID Example_SetRemapTable(HDC hdc)
{
Graphics graphics(hdc);
// Create an Image object based on a .bmp file.
// The image has one red stripe and one green stripe.
Image image(L"RedGreenStripes.bmp");
// Create an ImageAttributes object and set its remap table.
ImageAttributes imageAtt;
ColorMap cMap;
cMap.oldColor = Color(255, 255, 0, 0); // red
cMap.newColor = Color(255, 0, 0, 255); // blue
imageAtt.SetRemapTable(12, &cMap,
ColorAdjustTypeDefault);
// Draw the image with no color adjustment.
graphics.DrawImage(&image, 10, 10, image.GetWidth(), image.GetHeight());
// Draw the image with red converted to blue.
graphics.DrawImage(&image,
Rect(100, 10, image.GetWidth(), image.GetHeight()), // dest rect
0, 0, image.GetWidth(), image.GetHeight(), // source rect
UnitPixel,
&imageAtt);
}
The following illustration shows the output of the preceding code.
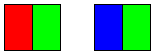
Requirements
Requirement | Value |
---|---|
Minimum supported client | Windows XP, Windows 2000 Professional [desktop apps only] |
Minimum supported server | Windows 2000 Server [desktop apps only] |
Target Platform | Windows |
Header | gdiplusimageattributes.h (include Gdiplus.h) |
Library | Gdiplus.lib |
DLL | Gdiplus.dll |
See also
Feedback
https://aka.ms/ContentUserFeedback.
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see:Submit and view feedback for