Metodo ID2D1RenderTarget::D rawRectangle(constD2D1_RECT_F&,ID2D1Brush*,FLOAT,ID2D1StrokeStyle*) (d2d1.h)
Disegna la struttura di un rettangolo con le dimensioni e lo stile del tratto specificati.
Sintassi
void DrawRectangle(
const D2D1_RECT_F & rect,
ID2D1Brush *brush,
FLOAT strokeWidth,
ID2D1StrokeStyle *strokeStyle
);
Parametri
rect
Tipo: [in] const D2D1_RECT_F &
Dimensioni del rettangolo da disegnare, in pixel indipendenti dal dispositivo.
brush
Tipo: [in] ID2D1Brush*
Pennello usato per disegnare il tratto del rettangolo.
strokeWidth
Tipo: [in] FLOAT
Larghezza del tratto, in pixel indipendenti dal dispositivo. Il valore deve essere maggiore o uguale a 0,0f. Se questo parametro non è specificato, il valore predefinito è 1.0f. Il tratto è centrato sulla linea.
strokeStyle
Tipo: [in, facoltativo] ID2D1StrokeStyle*
Stile del tratto da disegnare o NULL per disegnare un tratto solido.
Valore restituito
nessuno
Osservazioni
Quando questo metodo ha esito negativo, non restituisce un codice di errore. Per determinare se un metodo di disegno (ad esempio DrawRectangle) non è riuscito, controllare il risultato restituito dal metodo ID2D1RenderTarget::EndDraw o ID2D1RenderTarget::Flush .
Esempio
Nell'esempio seguente viene usato un ID2D1HwndRenderTarget per disegnare e riempire diversi rettangoli. In questo esempio viene generato l'output illustrato nella figura seguente.
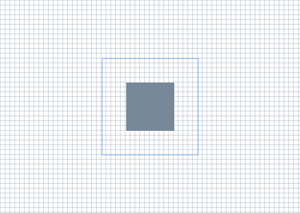
// This method discards device-specific
// resources if the Direct3D device disappears during execution and
// recreates the resources the next time it's invoked.
HRESULT DemoApp::OnRender()
{
HRESULT hr = S_OK;
hr = CreateDeviceResources();
if (SUCCEEDED(hr))
{
m_pRenderTarget->BeginDraw();
m_pRenderTarget->SetTransform(D2D1::Matrix3x2F::Identity());
m_pRenderTarget->Clear(D2D1::ColorF(D2D1::ColorF::White));
D2D1_SIZE_F rtSize = m_pRenderTarget->GetSize();
// Draw a grid background.
int width = static_cast<int>(rtSize.width);
int height = static_cast<int>(rtSize.height);
for (int x = 0; x < width; x += 10)
{
m_pRenderTarget->DrawLine(
D2D1::Point2F(static_cast<FLOAT>(x), 0.0f),
D2D1::Point2F(static_cast<FLOAT>(x), rtSize.height),
m_pLightSlateGrayBrush,
0.5f
);
}
for (int y = 0; y < height; y += 10)
{
m_pRenderTarget->DrawLine(
D2D1::Point2F(0.0f, static_cast<FLOAT>(y)),
D2D1::Point2F(rtSize.width, static_cast<FLOAT>(y)),
m_pLightSlateGrayBrush,
0.5f
);
}
// Draw two rectangles.
D2D1_RECT_F rectangle1 = D2D1::RectF(
rtSize.width/2 - 50.0f,
rtSize.height/2 - 50.0f,
rtSize.width/2 + 50.0f,
rtSize.height/2 + 50.0f
);
D2D1_RECT_F rectangle2 = D2D1::RectF(
rtSize.width/2 - 100.0f,
rtSize.height/2 - 100.0f,
rtSize.width/2 + 100.0f,
rtSize.height/2 + 100.0f
);
// Draw a filled rectangle.
m_pRenderTarget->FillRectangle(&rectangle1, m_pLightSlateGrayBrush);
// Draw the outline of a rectangle.
m_pRenderTarget->DrawRectangle(&rectangle2, m_pCornflowerBlueBrush);
hr = m_pRenderTarget->EndDraw();
}
if (hr == D2DERR_RECREATE_TARGET)
{
hr = S_OK;
DiscardDeviceResources();
}
return hr;
}
Per un'esercitazione correlata, vedere Creare un'applicazione Direct2D semplice.
Requisiti
Requisito | Valore |
---|---|
Client minimo supportato | Windows 7, Windows Vista con SP2 e Aggiornamento della piattaforma per Windows Vista [app desktop | App UWP] |
Server minimo supportato | Windows Server 2008 R2, Windows Server 2008 con SP2 e Platform Update per Windows Server 2008 [app desktop | App UWP] |
Piattaforma di destinazione | Windows |
Intestazione | d2d1.h |
Libreria | D2d1.lib |
DLL | D2d1.dll |
Vedi anche
Creare un'applicazione Direct2D semplice
Commenti e suggerimenti
https://aka.ms/ContentUserFeedback.
Presto disponibile: Nel corso del 2024 verranno gradualmente disattivati i problemi di GitHub come meccanismo di feedback per il contenuto e ciò verrà sostituito con un nuovo sistema di feedback. Per altre informazioni, vedereInvia e visualizza il feedback per