GestureRecognizer クラス
定義
重要
一部の情報は、リリース前に大きく変更される可能性があるプレリリースされた製品に関するものです。 Microsoft は、ここに記載されている情報について、明示または黙示を問わず、一切保証しません。
ジェスチャと操作の認識、イベント リスナー、設定を提供します。
public ref class GestureRecognizer sealed
/// [Windows.Foundation.Metadata.Activatable(65536, Windows.Foundation.UniversalApiContract)]
/// [Windows.Foundation.Metadata.ContractVersion(Windows.Foundation.UniversalApiContract, 65536)]
/// [Windows.Foundation.Metadata.MarshalingBehavior(Windows.Foundation.Metadata.MarshalingType.None)]
class GestureRecognizer final
/// [Windows.Foundation.Metadata.ContractVersion(Windows.Foundation.UniversalApiContract, 65536)]
/// [Windows.Foundation.Metadata.MarshalingBehavior(Windows.Foundation.Metadata.MarshalingType.None)]
/// [Windows.Foundation.Metadata.Activatable(65536, "Windows.Foundation.UniversalApiContract")]
class GestureRecognizer final
/// [Windows.Foundation.Metadata.ContractVersion(Windows.Foundation.UniversalApiContract, 65536)]
/// [Windows.Foundation.Metadata.MarshalingBehavior(Windows.Foundation.Metadata.MarshalingType.None)]
/// [Windows.Foundation.Metadata.Activatable(65536, "Windows.Foundation.UniversalApiContract")]
/// [Windows.Foundation.Metadata.Threading(Windows.Foundation.Metadata.ThreadingModel.Both)]
class GestureRecognizer final
[Windows.Foundation.Metadata.Activatable(65536, typeof(Windows.Foundation.UniversalApiContract))]
[Windows.Foundation.Metadata.ContractVersion(typeof(Windows.Foundation.UniversalApiContract), 65536)]
[Windows.Foundation.Metadata.MarshalingBehavior(Windows.Foundation.Metadata.MarshalingType.None)]
public sealed class GestureRecognizer
[Windows.Foundation.Metadata.ContractVersion(typeof(Windows.Foundation.UniversalApiContract), 65536)]
[Windows.Foundation.Metadata.MarshalingBehavior(Windows.Foundation.Metadata.MarshalingType.None)]
[Windows.Foundation.Metadata.Activatable(65536, "Windows.Foundation.UniversalApiContract")]
public sealed class GestureRecognizer
[Windows.Foundation.Metadata.ContractVersion(typeof(Windows.Foundation.UniversalApiContract), 65536)]
[Windows.Foundation.Metadata.MarshalingBehavior(Windows.Foundation.Metadata.MarshalingType.None)]
[Windows.Foundation.Metadata.Activatable(65536, "Windows.Foundation.UniversalApiContract")]
[Windows.Foundation.Metadata.Threading(Windows.Foundation.Metadata.ThreadingModel.Both)]
public sealed class GestureRecognizer
function GestureRecognizer()
Public NotInheritable Class GestureRecognizer
- 継承
- 属性
Windows の要件
デバイス ファミリ |
Windows 10 (10.0.10240.0 で導入)
|
API contract |
Windows.Foundation.UniversalApiContract (v1.0 で導入)
|
例
ここでは、ポインターとジェスチャの両方の入力を処理するための入力イベント ハンドラーのコレクションを使用して GestureRecognizer オブジェクトを設定します。 Windows ランタイム イベントをリッスンして処理する方法の詳細については、「イベントとルーティング イベントの概要」を参照してください。 完全な実装については、 基本的な入力サンプル を参照してください。
class ManipulationInputProcessor
{
GestureRecognizer recognizer;
UIElement element;
UIElement reference;
TransformGroup cumulativeTransform;
MatrixTransform previousTransform;
CompositeTransform deltaTransform;
public ManipulationInputProcessor(GestureRecognizer gestureRecognizer, UIElement target, UIElement referenceFrame)
{
recognizer = gestureRecognizer;
element = target;
reference = referenceFrame;
// Initialize the transforms that will be used to manipulate the shape
InitializeTransforms();
// The GestureSettings property dictates what manipulation events the
// Gesture Recognizer will listen to. This will set it to a limited
// subset of these events.
recognizer.GestureSettings = GenerateDefaultSettings();
// Set up pointer event handlers. These receive input events that are used by the gesture recognizer.
element.PointerPressed += OnPointerPressed;
element.PointerMoved += OnPointerMoved;
element.PointerReleased += OnPointerReleased;
element.PointerCanceled += OnPointerCanceled;
// Set up event handlers to respond to gesture recognizer output
recognizer.ManipulationStarted += OnManipulationStarted;
recognizer.ManipulationUpdated += OnManipulationUpdated;
recognizer.ManipulationCompleted += OnManipulationCompleted;
recognizer.ManipulationInertiaStarting += OnManipulationInertiaStarting;
}
public void InitializeTransforms()
{
cumulativeTransform = new TransformGroup();
deltaTransform = new CompositeTransform();
previousTransform = new MatrixTransform() { Matrix = Matrix.Identity };
cumulativeTransform.Children.Add(previousTransform);
cumulativeTransform.Children.Add(deltaTransform);
element.RenderTransform = cumulativeTransform;
}
// Return the default GestureSettings for this sample
GestureSettings GenerateDefaultSettings()
{
return GestureSettings.ManipulationTranslateX |
GestureSettings.ManipulationTranslateY |
GestureSettings.ManipulationRotate |
GestureSettings.ManipulationTranslateInertia |
GestureSettings.ManipulationRotateInertia;
}
// Route the pointer pressed event to the gesture recognizer.
// The points are in the reference frame of the canvas that contains the rectangle element.
void OnPointerPressed(object sender, PointerRoutedEventArgs args)
{
// Set the pointer capture to the element being interacted with so that only it
// will fire pointer-related events
element.CapturePointer(args.Pointer);
// Feed the current point into the gesture recognizer as a down event
recognizer.ProcessDownEvent(args.GetCurrentPoint(reference));
}
// Route the pointer moved event to the gesture recognizer.
// The points are in the reference frame of the canvas that contains the rectangle element.
void OnPointerMoved(object sender, PointerRoutedEventArgs args)
{
// Feed the set of points into the gesture recognizer as a move event
recognizer.ProcessMoveEvents(args.GetIntermediatePoints(reference));
}
// Route the pointer released event to the gesture recognizer.
// The points are in the reference frame of the canvas that contains the rectangle element.
void OnPointerReleased(object sender, PointerRoutedEventArgs args)
{
// Feed the current point into the gesture recognizer as an up event
recognizer.ProcessUpEvent(args.GetCurrentPoint(reference));
// Release the pointer
element.ReleasePointerCapture(args.Pointer);
}
// Route the pointer canceled event to the gesture recognizer.
// The points are in the reference frame of the canvas that contains the rectangle element.
void OnPointerCanceled(object sender, PointerRoutedEventArgs args)
{
recognizer.CompleteGesture();
element.ReleasePointerCapture(args.Pointer);
}
// When a manipulation begins, change the color of the object to reflect
// that a manipulation is in progress
void OnManipulationStarted(object sender, ManipulationStartedEventArgs e)
{
Border b = element as Border;
b.Background = new SolidColorBrush(Windows.UI.Colors.DeepSkyBlue);
}
// Process the change resulting from a manipulation
void OnManipulationUpdated(object sender, ManipulationUpdatedEventArgs e)
{
previousTransform.Matrix = cumulativeTransform.Value;
// Get the center point of the manipulation for rotation
Point center = new Point(e.Position.X, e.Position.Y);
deltaTransform.CenterX = center.X;
deltaTransform.CenterY = center.Y;
// Look at the Delta property of the ManipulationDeltaRoutedEventArgs to retrieve
// the rotation, X, and Y changes
deltaTransform.Rotation = e.Delta.Rotation;
deltaTransform.TranslateX = e.Delta.Translation.X;
deltaTransform.TranslateY = e.Delta.Translation.Y;
}
// When a manipulation that's a result of inertia begins, change the color of the
// the object to reflect that inertia has taken over
void OnManipulationInertiaStarting(object sender, ManipulationInertiaStartingEventArgs e)
{
Border b = element as Border;
b.Background = new SolidColorBrush(Windows.UI.Colors.RoyalBlue);
}
// When a manipulation has finished, reset the color of the object
void OnManipulationCompleted(object sender, ManipulationCompletedEventArgs e)
{
Border b = element as Border;
b.Background = new SolidColorBrush(Windows.UI.Colors.LightGray);
}
// Modify the GestureSettings property to only allow movement on the X axis
public void LockToXAxis()
{
recognizer.CompleteGesture();
recognizer.GestureSettings |= GestureSettings.ManipulationTranslateY | GestureSettings.ManipulationTranslateX;
recognizer.GestureSettings ^= GestureSettings.ManipulationTranslateY;
}
// Modify the GestureSettings property to only allow movement on the Y axis
public void LockToYAxis()
{
recognizer.CompleteGesture();
recognizer.GestureSettings |= GestureSettings.ManipulationTranslateY | GestureSettings.ManipulationTranslateX;
recognizer.GestureSettings ^= GestureSettings.ManipulationTranslateX;
}
// Modify the GestureSettings property to allow movement on both the X and Y axes
public void MoveOnXAndYAxes()
{
recognizer.CompleteGesture();
recognizer.GestureSettings |= GestureSettings.ManipulationTranslateX | GestureSettings.ManipulationTranslateY;
}
// Modify the GestureSettings property to enable or disable inertia based on the passed-in value
public void UseInertia(bool inertia)
{
if (!inertia)
{
recognizer.CompleteGesture();
recognizer.GestureSettings ^= GestureSettings.ManipulationTranslateInertia | GestureSettings.ManipulationRotateInertia;
}
else
{
recognizer.GestureSettings |= GestureSettings.ManipulationTranslateInertia | GestureSettings.ManipulationRotateInertia;
}
}
public void Reset()
{
element.RenderTransform = null;
recognizer.CompleteGesture();
InitializeTransforms();
recognizer.GestureSettings = GenerateDefaultSettings();
}
}
サンプル
アーカイブされたサンプル
注釈
アプリの起動時に、適切な要素ごとにジェスチャ オブジェクトを作成できます。 ただし、作成する必要があるジェスチャ オブジェクトの数 (数百個のジグソーパズルなど) によっては、この方法は適切にスケーリングされない場合があります。
この場合、 ポインターダウン イベントでジェスチャ オブジェクトを動的に作成し、 MSGestureEnd イベントで破棄できます。 この方法は適切にスケーリングされますが、これらのオブジェクトの作成と解放によりオーバーヘッドが発生します。
または、再利用可能なジェスチャ オブジェクトのプールを静的に割り当てて動的に管理することもできます。
注意
このクラスはアジャイルではありません。つまり、スレッド モデルとマーシャリング動作を考慮する必要があります。 詳細については、「スレッド処理とマーシャリング (C++/CX)」および「マルチスレッド環境でのWindows ランタイム オブジェクトの使用 (.NET)」を参照してください。
クロススライド機能の使用方法の詳細については、「クロススライド のガイドライン」を参照してください。 クロススライド操作で使われるしきい値の距離を次の図に示します。
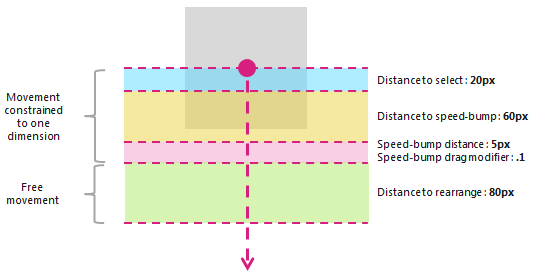
PivotRadius プロパティと PivotCenter プロパティは、単一ポインター入力が検出された場合にのみ使用されます。 複数のポインター入力には影響しません。 これらのプロパティの値は、対話中に定期的に更新する必要があります。
回転は、 manipulationRotate が GestureSettings プロパティを使用して設定されている場合にのみ、GestureRecognizer によってサポートされます。
PivotRadius の値が 0 に設定されている場合、単一ポインター入力では回転はサポートされません。
バージョン履歴
Windows のバージョン | SDK バージョン | 追加された値 |
---|---|---|
2004 | 19041 | HoldMaxContactCount |
2004 | 19041 | HoldMinContactCount |
2004 | 19041 | HoldRadius |
2004 | 19041 | HoldStartDelay |
2004 | 19041 | TapMaxContactCount |
2004 | 19041 | TapMinContactCount |
2004 | 19041 | TranslationMaxContactCount |
2004 | 19041 | TranslationMinContactCount |
コンストラクター
GestureRecognizer() |
GestureRecognizer オブジェクトの新しいインスタンスを初期化します。 |
プロパティ
AutoProcessInertia |
慣性時の操作が自動的に生成されるかどうかを示す値を取得または設定します。 |
CrossSlideExact |
スライド間操作の最初の接触から終了までの正確な距離を報告するかどうかを示す値を取得または設定します。既定では、クロススライド操作のためにシステムによって報告された最初の位置から小さな距離のしきい値が減算されます。 このフラグが設定されている場合、距離のしきい値は初期位置から減算されません。 注意 この距離しきい値は、初期検出後の接触のわずかな動きを考慮するためのものです。 これは、システムがクロススライディングとパンを区別するのに役立ち、タップ ジェスチャがどちらとしても解釈されないようにするのに役立ちます。 |
CrossSlideHorizontally |
クロススライド軸が水平かどうかを示す値を取得または設定します。 |
CrossSlideThresholds |
CrossSliding 相互作用の距離しきい値を示す値を取得または設定します。 |
GestureSettings |
アプリケーションでサポートされているジェスチャと操作の設定を示す値を取得または設定します。 |
HoldMaxContactCount |
Windows.UI.Input.GestureRecognizer.Holding イベントを認識するために必要な連絡先ポイントの最大数を取得または設定します。 |
HoldMinContactCount |
Windows.UI.Input.GestureRecognizer.Holding イベントを認識するために必要な連絡先ポイントの最小数を取得または設定します。 |
HoldRadius |
Windows.UI.Input.GestureRecognizer.Holding イベントで認識される連絡先ポイントの半径を取得または設定します。 |
HoldStartDelay |
Windows.UI.Input.GestureRecognizer.Holding イベントに対して連絡先が認識される時間のしきい値を取得または設定します。 |
InertiaExpansion |
慣性の開始から慣性終了までのオブジェクトのサイズの相対的な変化を示す値を取得または設定します (サイズ変更またはスケーリングが完了したとき)。 |
InertiaExpansionDeceleration |
慣性の開始から慣性終了までの減速率を示す値を取得または設定します (サイズ変更または拡張操作が完了した場合)。 |
InertiaRotationAngle |
慣性の最後のオブジェクトの回転角度 (回転操作が完了したとき) を示す値を取得または設定します。 |
InertiaRotationDeceleration |
慣性の開始から慣性終了までの減速率 (回転操作が完了したとき) を示す値を取得または設定します。 |
InertiaTranslationDeceleration |
慣性の開始から慣性終了までの減速率を示す値を取得または設定します (変換操作が完了したとき)。 |
InertiaTranslationDisplacement |
慣性の開始から慣性終了までのオブジェクトの画面位置の相対的な変化を示す値を取得または設定します (変換操作が完了したとき)。 |
IsActive |
相互作用が処理されているかどうかを示す値を取得します。 |
IsInertial |
慣性中に操作がまだ処理されているかどうかを示す値を取得します (入力ポイントがアクティブでない)。 |
ManipulationExact |
最初の接触から操作の終了までの正確な距離を報告するかどうかを示す値を取得または設定します。 既定では、システムによって報告された最初のデルタから小さな距離のしきい値が減算されます。 この距離しきい値は、タップ ジェスチャを処理する際の接触のわずかな動きを考慮するためのものです。 このフラグが設定されている場合、距離のしきい値は最初のデルタから減算されません。 |
MouseWheelParameters |
マウス デバイスのホイール ボタンに関連付けられているプロパティのセットを取得します。 |
PivotCenter |
単一ポインター入力が検出された場合の回転操作の中心点を取得または設定します。 |
PivotRadius |
単一ポインター入力が検出されたときに回転操作を行うために、 ピボットセンター からポインター入力までの半径を取得または設定します。 |
ShowGestureFeedback |
対話中に視覚的フィードバックを表示するかどうかを示す値を取得または設定します。 |
TapMaxContactCount |
Windows.UI.Input.GestureRecognizer.Tapped イベントを認識するために必要な連絡先ポイントの最大数を取得または設定します。 |
TapMinContactCount |
Windows.UI.Input.GestureRecognizer.Tapped イベントを認識するために必要な連絡先ポイントの最小数を取得または設定します。 |
TranslationMaxContactCount |
翻訳 (またはパン) イベントを認識するために必要な連絡先ポイントの最大数を取得または設定します。 |
TranslationMinContactCount |
翻訳 (またはパン) イベントを認識するために必要な連絡先ポイントの最小数を取得または設定します。 |
メソッド
CanBeDoubleTap(PointerPoint) |
ダブルタップ ジェスチャの 2 回目のタップとしてタップを引き続き解釈できるかどうかを識別します。 |
CompleteGesture() |
ジェスチャ認識エンジンが対話を終了させます。 |
ProcessDownEvent(PointerPoint) |
ポインター入力を処理し、GestureSettings プロパティで指定されたジェスチャと操作のポインターダウン アクションに適した GestureRecognizer イベントを発生させます。 |
ProcessInertia() |
慣性計算を実行し、さまざまな慣性イベントを発生させます。 |
ProcessMouseWheelEvent(PointerPoint, Boolean, Boolean) |
ポインター入力を処理し、GestureSettings プロパティで指定されたジェスチャと操作のマウス ホイール アクションに適した GestureRecognizer イベントを発生させます。 |
ProcessMoveEvents(IVector<PointerPoint>) |
ポインター入力を処理し、GestureSettings プロパティで指定されたジェスチャと操作のポインター移動アクションに適した GestureRecognizer イベントを発生させます。 |
ProcessUpEvent(PointerPoint) |
ポインター入力を処理し、GestureSettings プロパティで指定されたジェスチャと操作のポインターアップ アクションに適した GestureRecognizer イベントを発生させます。 |
イベント
CrossSliding |
ユーザーが、1 つの軸に沿ったパンのみをサポートするコンテンツ領域内で (1 回のタッチ接触を介して) スライド または スワイプ ジェスチャを実行したときに発生します。 ジェスチャは、このパン軸に対して垂直な方向で行う必要があります。 注意 スワイプは短いスライディング ジェスチャであり、長いスライド ジェスチャが距離しきい値を超えて再配置アクションが行われる間に、選択アクションが実行されます。 スワイプジェスチャとスライド ジェスチャを次の図に示します。
|
Dragging |
ユーザーがマウスまたはペン/スタイラス (単一の連絡先) を使用して スライド または スワイプ ジェスチャを実行すると発生します。 |
Holding |
ユーザーが (シングル タッチ、マウス、またはペン/スタイラスの接触を使用して) 長押しジェスチャを実行すると発生します。 |
ManipulationCompleted |
入力ポイントが持ち上げられ、慣性による後続のすべてのモーション (平行移動、展開、または回転) が終了したときに発生します。 |
ManipulationInertiaStarting |
操作中にすべての接触点が持ち上げられ、操作の速度が慣性動作を開始するのに十分な大きさである場合に発生します (入力ポインターがリフトされた後も移動、拡張、または回転が続行されます)。 |
ManipulationStarted |
1 つ以上の入力ポイントが開始され、後続のモーション (平行移動、展開、または回転) が開始されたときに発生します。 |
ManipulationUpdated |
1 つ以上の入力ポイントが開始され、その後のモーション (平行移動、展開、または回転) が進行中の後に発生します。 |
RightTapped |
入力デバイスに関係なく、ポインター入力が右タップ ジェスチャとして解釈されるときに発生します。
|
Tapped |
ポインター入力がタップ ジェスチャとして解釈されるときに発生します。 |
適用対象
こちらもご覧ください
フィードバック
https://aka.ms/ContentUserFeedback」を参照してください。
以下は間もなく提供いたします。2024 年を通じて、コンテンツのフィードバック メカニズムとして GitHub の issue を段階的に廃止し、新しいフィードバック システムに置き換えます。 詳細については、「フィードバックの送信と表示