GestureRecognizer 類別
定義
重要
部分資訊涉及發行前產品,在發行之前可能會有大幅修改。 Microsoft 對此處提供的資訊,不做任何明確或隱含的瑕疵擔保。
提供手勢和操作辨識、事件接聽程式和設定。
public ref class GestureRecognizer sealed
/// [Windows.Foundation.Metadata.Activatable(65536, Windows.Foundation.UniversalApiContract)]
/// [Windows.Foundation.Metadata.ContractVersion(Windows.Foundation.UniversalApiContract, 65536)]
/// [Windows.Foundation.Metadata.MarshalingBehavior(Windows.Foundation.Metadata.MarshalingType.None)]
class GestureRecognizer final
/// [Windows.Foundation.Metadata.ContractVersion(Windows.Foundation.UniversalApiContract, 65536)]
/// [Windows.Foundation.Metadata.MarshalingBehavior(Windows.Foundation.Metadata.MarshalingType.None)]
/// [Windows.Foundation.Metadata.Activatable(65536, "Windows.Foundation.UniversalApiContract")]
class GestureRecognizer final
/// [Windows.Foundation.Metadata.ContractVersion(Windows.Foundation.UniversalApiContract, 65536)]
/// [Windows.Foundation.Metadata.MarshalingBehavior(Windows.Foundation.Metadata.MarshalingType.None)]
/// [Windows.Foundation.Metadata.Activatable(65536, "Windows.Foundation.UniversalApiContract")]
/// [Windows.Foundation.Metadata.Threading(Windows.Foundation.Metadata.ThreadingModel.Both)]
class GestureRecognizer final
[Windows.Foundation.Metadata.Activatable(65536, typeof(Windows.Foundation.UniversalApiContract))]
[Windows.Foundation.Metadata.ContractVersion(typeof(Windows.Foundation.UniversalApiContract), 65536)]
[Windows.Foundation.Metadata.MarshalingBehavior(Windows.Foundation.Metadata.MarshalingType.None)]
public sealed class GestureRecognizer
[Windows.Foundation.Metadata.ContractVersion(typeof(Windows.Foundation.UniversalApiContract), 65536)]
[Windows.Foundation.Metadata.MarshalingBehavior(Windows.Foundation.Metadata.MarshalingType.None)]
[Windows.Foundation.Metadata.Activatable(65536, "Windows.Foundation.UniversalApiContract")]
public sealed class GestureRecognizer
[Windows.Foundation.Metadata.ContractVersion(typeof(Windows.Foundation.UniversalApiContract), 65536)]
[Windows.Foundation.Metadata.MarshalingBehavior(Windows.Foundation.Metadata.MarshalingType.None)]
[Windows.Foundation.Metadata.Activatable(65536, "Windows.Foundation.UniversalApiContract")]
[Windows.Foundation.Metadata.Threading(Windows.Foundation.Metadata.ThreadingModel.Both)]
public sealed class GestureRecognizer
function GestureRecognizer()
Public NotInheritable Class GestureRecognizer
- 繼承
- 屬性
Windows 需求
裝置系列 |
Windows 10 (已於 10.0.10240.0 引進)
|
API contract |
Windows.Foundation.UniversalApiContract (已於 v1.0 引進)
|
範例
在這裡,我們會使用輸入事件處理常式集合來設定 GestureRecognizer 物件,以處理指標和手勢輸入。 如需如何接聽和處理Windows 執行階段事件的詳細資訊,請參閱事件和路由事件概觀。 如需完整實作,請參閱 基本輸入範例 。
class ManipulationInputProcessor
{
GestureRecognizer recognizer;
UIElement element;
UIElement reference;
TransformGroup cumulativeTransform;
MatrixTransform previousTransform;
CompositeTransform deltaTransform;
public ManipulationInputProcessor(GestureRecognizer gestureRecognizer, UIElement target, UIElement referenceFrame)
{
recognizer = gestureRecognizer;
element = target;
reference = referenceFrame;
// Initialize the transforms that will be used to manipulate the shape
InitializeTransforms();
// The GestureSettings property dictates what manipulation events the
// Gesture Recognizer will listen to. This will set it to a limited
// subset of these events.
recognizer.GestureSettings = GenerateDefaultSettings();
// Set up pointer event handlers. These receive input events that are used by the gesture recognizer.
element.PointerPressed += OnPointerPressed;
element.PointerMoved += OnPointerMoved;
element.PointerReleased += OnPointerReleased;
element.PointerCanceled += OnPointerCanceled;
// Set up event handlers to respond to gesture recognizer output
recognizer.ManipulationStarted += OnManipulationStarted;
recognizer.ManipulationUpdated += OnManipulationUpdated;
recognizer.ManipulationCompleted += OnManipulationCompleted;
recognizer.ManipulationInertiaStarting += OnManipulationInertiaStarting;
}
public void InitializeTransforms()
{
cumulativeTransform = new TransformGroup();
deltaTransform = new CompositeTransform();
previousTransform = new MatrixTransform() { Matrix = Matrix.Identity };
cumulativeTransform.Children.Add(previousTransform);
cumulativeTransform.Children.Add(deltaTransform);
element.RenderTransform = cumulativeTransform;
}
// Return the default GestureSettings for this sample
GestureSettings GenerateDefaultSettings()
{
return GestureSettings.ManipulationTranslateX |
GestureSettings.ManipulationTranslateY |
GestureSettings.ManipulationRotate |
GestureSettings.ManipulationTranslateInertia |
GestureSettings.ManipulationRotateInertia;
}
// Route the pointer pressed event to the gesture recognizer.
// The points are in the reference frame of the canvas that contains the rectangle element.
void OnPointerPressed(object sender, PointerRoutedEventArgs args)
{
// Set the pointer capture to the element being interacted with so that only it
// will fire pointer-related events
element.CapturePointer(args.Pointer);
// Feed the current point into the gesture recognizer as a down event
recognizer.ProcessDownEvent(args.GetCurrentPoint(reference));
}
// Route the pointer moved event to the gesture recognizer.
// The points are in the reference frame of the canvas that contains the rectangle element.
void OnPointerMoved(object sender, PointerRoutedEventArgs args)
{
// Feed the set of points into the gesture recognizer as a move event
recognizer.ProcessMoveEvents(args.GetIntermediatePoints(reference));
}
// Route the pointer released event to the gesture recognizer.
// The points are in the reference frame of the canvas that contains the rectangle element.
void OnPointerReleased(object sender, PointerRoutedEventArgs args)
{
// Feed the current point into the gesture recognizer as an up event
recognizer.ProcessUpEvent(args.GetCurrentPoint(reference));
// Release the pointer
element.ReleasePointerCapture(args.Pointer);
}
// Route the pointer canceled event to the gesture recognizer.
// The points are in the reference frame of the canvas that contains the rectangle element.
void OnPointerCanceled(object sender, PointerRoutedEventArgs args)
{
recognizer.CompleteGesture();
element.ReleasePointerCapture(args.Pointer);
}
// When a manipulation begins, change the color of the object to reflect
// that a manipulation is in progress
void OnManipulationStarted(object sender, ManipulationStartedEventArgs e)
{
Border b = element as Border;
b.Background = new SolidColorBrush(Windows.UI.Colors.DeepSkyBlue);
}
// Process the change resulting from a manipulation
void OnManipulationUpdated(object sender, ManipulationUpdatedEventArgs e)
{
previousTransform.Matrix = cumulativeTransform.Value;
// Get the center point of the manipulation for rotation
Point center = new Point(e.Position.X, e.Position.Y);
deltaTransform.CenterX = center.X;
deltaTransform.CenterY = center.Y;
// Look at the Delta property of the ManipulationDeltaRoutedEventArgs to retrieve
// the rotation, X, and Y changes
deltaTransform.Rotation = e.Delta.Rotation;
deltaTransform.TranslateX = e.Delta.Translation.X;
deltaTransform.TranslateY = e.Delta.Translation.Y;
}
// When a manipulation that's a result of inertia begins, change the color of the
// the object to reflect that inertia has taken over
void OnManipulationInertiaStarting(object sender, ManipulationInertiaStartingEventArgs e)
{
Border b = element as Border;
b.Background = new SolidColorBrush(Windows.UI.Colors.RoyalBlue);
}
// When a manipulation has finished, reset the color of the object
void OnManipulationCompleted(object sender, ManipulationCompletedEventArgs e)
{
Border b = element as Border;
b.Background = new SolidColorBrush(Windows.UI.Colors.LightGray);
}
// Modify the GestureSettings property to only allow movement on the X axis
public void LockToXAxis()
{
recognizer.CompleteGesture();
recognizer.GestureSettings |= GestureSettings.ManipulationTranslateY | GestureSettings.ManipulationTranslateX;
recognizer.GestureSettings ^= GestureSettings.ManipulationTranslateY;
}
// Modify the GestureSettings property to only allow movement on the Y axis
public void LockToYAxis()
{
recognizer.CompleteGesture();
recognizer.GestureSettings |= GestureSettings.ManipulationTranslateY | GestureSettings.ManipulationTranslateX;
recognizer.GestureSettings ^= GestureSettings.ManipulationTranslateX;
}
// Modify the GestureSettings property to allow movement on both the X and Y axes
public void MoveOnXAndYAxes()
{
recognizer.CompleteGesture();
recognizer.GestureSettings |= GestureSettings.ManipulationTranslateX | GestureSettings.ManipulationTranslateY;
}
// Modify the GestureSettings property to enable or disable inertia based on the passed-in value
public void UseInertia(bool inertia)
{
if (!inertia)
{
recognizer.CompleteGesture();
recognizer.GestureSettings ^= GestureSettings.ManipulationTranslateInertia | GestureSettings.ManipulationRotateInertia;
}
else
{
recognizer.GestureSettings |= GestureSettings.ManipulationTranslateInertia | GestureSettings.ManipulationRotateInertia;
}
}
public void Reset()
{
element.RenderTransform = null;
recognizer.CompleteGesture();
InitializeTransforms();
recognizer.GestureSettings = GenerateDefaultSettings();
}
}
範例
封存範例
備註
當您的應用程式啟動時,您可以為每個適當的元素建立手勢物件。 不過,根據您需要建立 (的手勢物件數目而定,此方法可能無法妥善調整,例如,有數百個片段) 的抖動問題。
在此情況下,您可以在 pointerdown 事件上動態建立手勢物件,並在 MSGestureEnd 事件上終結它們。 此方法會妥善調整,但會產生一些額外負荷,因為建立和釋放這些物件。
或者,您可以靜態配置並動態管理可重複使用手勢物件的集區。
注意
這個類別不是敏捷式的,這表示您需要考慮其執行緒模型和封送處理行為。 如需詳細資訊,請參閱執行緒和封送處理 (C++/CX) 和在多執行緒環境中使用 Windows 執行階段 物件 (.NET) 。
如需如何使用跨投影片功能的詳細資訊,請參閱 跨投影片的指導方針。 交叉滑動互動所使用的閾值距離如下圖所示。
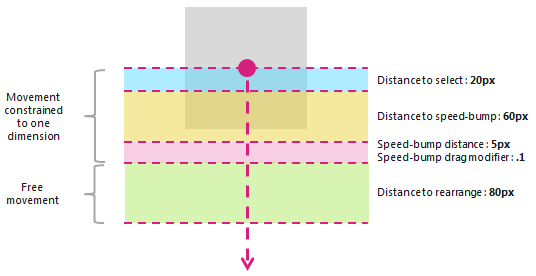
只有在偵測到單一指標輸入時,才會使用 PivotRadius 和 PivotCenter 屬性。 它們不會影響多個指標輸入。 這些屬性的值應該在互動期間定期更新。
只有當 manipulationRotate 是透過 GestureSettings 屬性設定時,GestureRecognizer 才支援旋轉。
如果 PivotRadius 的值設定為 0,則不支援單一指標輸入的旋轉。
版本歷程記錄
Windows 版本 | SDK 版本 | 新增值 |
---|---|---|
2004 | 19041 | HoldMaxContactCount |
2004 | 19041 | HoldMinContactCount |
2004 | 19041 | HoldRadius |
2004 | 19041 | HoldStartDelay |
2004 | 19041 | TapMaxContactCount |
2004 | 19041 | TapMinContactCount |
2004 | 19041 | TranslationMaxContactCount |
2004 | 19041 | TranslationMinContactCount |
建構函式
GestureRecognizer() |
初始化 GestureRecognizer 物件的新實例。 |
屬性
AutoProcessInertia |
取得或設定值,這個值表示是否自動產生慣性期間的操作。 |
CrossSlideExact |
取得或設定值,這個值表示是否報告從初始接觸到交叉投影片互動結尾的確切距離。根據預設,系統會從系統回報的第一個位置減去小型距離閾值,以進行跨投影片互動。 如果設定此旗標,則不會從初始位置減去距離臨界值。 注意 此距離臨界值旨在考慮初始偵測之後連絡人的任何稍微移動。 它可協助系統區分交叉滑動和移動流覽,並協助確保點選手勢不會解譯為任一種。 |
CrossSlideHorizontally |
取得或設定值,這個值表示交叉投影片軸是否為水準軸。 |
CrossSlideThresholds |
取得或設定值,指出 CrossSliding 互動的距離臨界值。 |
GestureSettings |
取得或設定值,這個值表示應用程式支援的手勢和操作設定。 |
HoldMaxContactCount |
取得或設定辨識 Windows.UI.Input.GestureRecognizer.Holding 事件所需的連絡點數目上限。 |
HoldMinContactCount |
取得或設定辨識 Windows.UI.Input.GestureRecognizer.Holding 事件所需的連絡點數目下限。 |
HoldRadius |
取得或設定針對 Windows.UI.Input.GestureRecognizer.Holding 事件辨識的連絡點半徑。 |
HoldStartDelay |
取得或設定 Windows.UI.Input.GestureRecognizer.Holding 事件可辨識連絡人的時間臨界值。 |
InertiaExpansion |
取得或設定值,指出調整大小或調整時,物件大小從慣性開頭到慣性結束 (的相對變更) 。 |
InertiaExpansionDeceleration |
取得或設定值,指出調整大小或展開時,操作完成) 時,從慣性開始到慣性結束 (的減速速率。 |
InertiaRotationAngle |
取得或設定值,這個值表示當旋轉操作完成) 時,在慣性 (結束之物件旋轉的最終角度。 |
InertiaRotationDeceleration |
取得或設定值,這個值表示旋轉操作完成) 時,從慣性開始到慣性結束 (的減速速率。 |
InertiaTranslationDeceleration |
取得或設定值,指出翻譯操作完成) 時,從慣性開始到慣性結束 (的減速速率。 |
InertiaTranslationDisplacement |
取得或設定值,指出當翻譯操作) 完成時,從慣性開始到慣性結束 (物件畫面位置的相對變更。 |
IsActive |
取得值,這個值表示是否正在處理互動。 |
IsInertial |
取得值,這個值表示操作是否仍在慣性期間處理, (沒有輸入點作用中) 。 |
ManipulationExact |
取得或設定值,這個值表示是否報告從初始接觸到互動結尾的確切距離。 根據預設,系統會從系統所報告的第一個差異減去小距離閾值。 此距離閾值旨在考慮在處理點選手勢時稍微移動連絡人。 如果設定此旗標,則不會從第一個差異減去距離臨界值。 |
MouseWheelParameters |
取得一組與滑鼠裝置的滾輪按鈕相關聯的屬性。 |
PivotCenter |
取得或設定偵測到單一指標輸入時旋轉互動的中心點。 |
PivotRadius |
取得或設定從 PivotCenter 到指標輸入的半徑,以在偵測到單一指標輸入時進行旋轉互動。 |
ShowGestureFeedback |
取得或設定值,指出在互動期間是否顯示視覺回饋。 |
TapMaxContactCount |
取得或設定辨識 Windows.UI.Input.GestureRecognizer.Tapped 事件所需的連絡點數目上限。 |
TapMinContactCount |
取得或設定辨識 Windows.UI.Input.GestureRecognizer.Tapped 事件所需的連絡點數目下限。 |
TranslationMaxContactCount |
取得或設定辨識翻譯 (或移動流覽) 事件所需的連絡人點數目上限。 |
TranslationMinContactCount |
取得或設定辨識翻譯 (或移動流覽) 事件所需的連絡點數目下限。 |
方法
CanBeDoubleTap(PointerPoint) |
識別點選是否仍可解譯為按兩下手勢的第二個點選。 |
CompleteGesture() |
讓手勢辨識器完成互動。 |
ProcessDownEvent(PointerPoint) |
處理指標輸入,並引發適用于GestureSettings屬性所指定手勢和操作的指標向下動作的GestureRecognizer事件。 |
ProcessInertia() |
執行慣性計算,並引發各種慣性事件。 |
ProcessMouseWheelEvent(PointerPoint, Boolean, Boolean) |
處理指標輸入,並引發適用于GestureSettings屬性所指定手勢和操作的滑鼠滾輪動作的GestureRecognizer事件。 |
ProcessMoveEvents(IVector<PointerPoint>) |
處理指標輸入,並引發適用于GestureSettings屬性所指定手勢和操作的指標移動動作的GestureRecognizer事件。 |
ProcessUpEvent(PointerPoint) |
處理指標輸入,並引發適用于GestureSettings屬性所指定手勢和操作指標向上動作的GestureRecognizer事件。 |
事件
CrossSliding |
發生于使用者在僅支援沿著單一軸移動流覽的內容區域內,透過單一觸控連絡人 () 執行 投影片 或 撥 動手勢時。 筆勢必須以垂直于這個移動流覽軸的方向發生。 注意 撥動是一個短滑動手勢,會導致選取動作,而較長的滑動手勢會跨越距離閾值,並導致重新排列動作。 下圖示范撥動和滑動手勢。
|
Dragging | |
Holding |
當使用者使用單一觸控、滑鼠或手寫筆接觸) 執行按住手勢 (時發生。 |
ManipulationCompleted |
當輸入點隨即提升,且所有後續動作 (轉譯、擴充或旋轉) 已結束時發生。 |
ManipulationInertiaStarting |
發生于操作期間所有接觸點的增益,而且操作速度足以在輸入指標隨即隨即) 之後起始慣性行為 (轉、擴充或旋轉。 |
ManipulationStarted |
發生于起始一或多個輸入點,而後續動作 (轉譯、展開或旋轉) 開始時。 |
ManipulationUpdated |
在起始一或多個輸入點之後發生,後續動作 (轉譯、擴充或旋轉) 正在進行中。 |
RightTapped |
無論輸入裝置為何,指標輸入都會解譯為右鍵手勢時發生。
|
Tapped |
發生于指標輸入解譯為點選手勢時。 |
適用於
另請參閱
意見反應
https://aka.ms/ContentUserFeedback。
即將登場:在 2024 年,我們將逐步淘汰 GitHub 問題作為內容的意見反應機制,並將它取代為新的意見反應系統。 如需詳細資訊,請參閱:提交並檢視相關的意見反應